목차
- 10 Best Python Techniques to Boost Efficiency in Automation
- 1. Web Scraping for Automated Data Collection
- Extracting Information from Websites
- 2. Automating Data Processing with Pandas
- Efficient Data Manipulation and Analysis
- 3. Web Browser Automation Using Selenium
- Automating Web Navigation and Interaction
- 4. Managing Files and Folders Automatically
- Organizing and Moving Files with Python
- 5. Sending Automated Emails and Notifications
- Streamlining Email Communication
- 6. Scheduling Tasks Automatically
- Running Scripts at Fixed Intervals
- 7. Automating Excel Reports and Data Processing
- Editing Excel Files Programmatically
- 8. Automating API Calls for Data Retrieval
- Fetching and Processing API Data
- 9. Image and Video Processing Automation
- Enhancing Images and Videos with Python
- 10. AI and Chatbot Automation
- Automating Responses with AI Models
- Conclusion
반응형
10 Best Python Techniques to Boost Efficiency in Automation
Discover the 10 most effective Python techniques for automating tasks, saving time, and increasing efficiency. Learn how to handle web scraping, file management, scheduling, and AI automation with real-world examples.
1. Web Scraping for Automated Data Collection
Extracting Information from Websites
- Python libraries like BeautifulSoup and requests help extract data from websites automatically.
- Automating data retrieval eliminates the need for manual copy-pasting and speeds up analysis.
Example Code:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
titles = soup.find_all("h2")
for title in titles:
print(title.text)
2. Automating Data Processing with Pandas
Efficient Data Manipulation and Analysis
- pandas helps automate data cleansing, transformation, and aggregation.
Example Code:
import pandas as pd
df = pd.read_csv("data.csv")
df["adjusted_value"] = df["column_name"] * 1.5 # Data transformation
df.to_csv("processed_data.csv", index=False)
3. Web Browser Automation Using Selenium
Automating Web Navigation and Interaction
- Selenium allows automation of website navigation, form filling, and login processes.
Example Code:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com/login")
username = driver.find_element("name", "username")
password = driver.find_element("name", "password")
username.send_keys("user123")
password.send_keys("mypassword")
login_button = driver.find_element("id", "login")
login_button.click()
4. Managing Files and Folders Automatically
Organizing and Moving Files with Python
- os and shutil modules help automate file management.
Example Code:
import os
import shutil
source_folder = "C:/Users/Desktop/source"
destination_folder = "C:/Users/Desktop/destination"
for filename in os.listdir(source_folder):
if filename.endswith(".txt"):
shutil.move(os.path.join(source_folder, filename), destination_folder)
5. Sending Automated Emails and Notifications
Streamlining Email Communication
- Use smtplib and email.mime to send automated emails.
Example Code:
import smtplib
from email.mime.text import MIMEText
sender = "your_email@gmail.com"
receiver = "receiver_email@gmail.com"
password = "your_password"
msg = MIMEText("This is an automated message.")
msg["Subject"] = "Automation Alert"
msg["From"] = sender
msg["To"] = receiver
server = smtplib.SMTP_SSL("smtp.gmail.com", 465)
server.login(sender, password)
server.sendmail(sender, receiver, msg.as_string())
server.quit()
6. Scheduling Tasks Automatically
Running Scripts at Fixed Intervals
- The schedule library allows execution of tasks at specific time intervals.
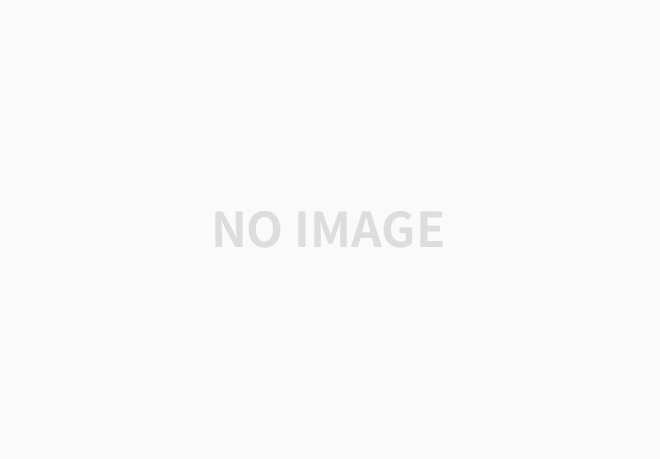
Example Code:
import schedule
import time
def job():
print("Scheduled task executed!")
schedule.every().hour.do(job)
while True:
schedule.run_pending()
time.sleep(1)
7. Automating Excel Reports and Data Processing
Editing Excel Files Programmatically
- Use openpyxl to automate Excel file updates.
Example Code:
import openpyxl
wb = openpyxl.load_workbook("report.xlsx")
sheet = wb.active
sheet["A1"] = "Updated Value"
wb.save("updated_report.xlsx")
8. Automating API Calls for Data Retrieval
Fetching and Processing API Data
- The requests library helps automate API data extraction.
Example Code:
import requests
api_url = "https://api.example.com/data"
response = requests.get(api_url)
if response.status_code == 200:
print(response.json())
9. Image and Video Processing Automation
Enhancing Images and Videos with Python
- Use Pillow and OpenCV to automate image transformations.
Example Code:
from PIL import Image
img = Image.open("image.jpg")
img = img.resize((300, 300))
img.save("resized_image.jpg")
10. AI and Chatbot Automation
Automating Responses with AI Models
- Use OpenAI’s API to integrate AI-driven chatbots.
Example Code:
import openai
openai.api_key = "your-api-key"
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "user", "content": "Hello, how can you help me?"}]
)
print(response["choices"][0]["message"]["content"])
Conclusion
Python automation simplifies complex tasks, enhances productivity, and reduces manual work. Whether you’re automating web scraping, file management, or AI-driven tasks, these techniques will streamline your workflow and save time.
반응형